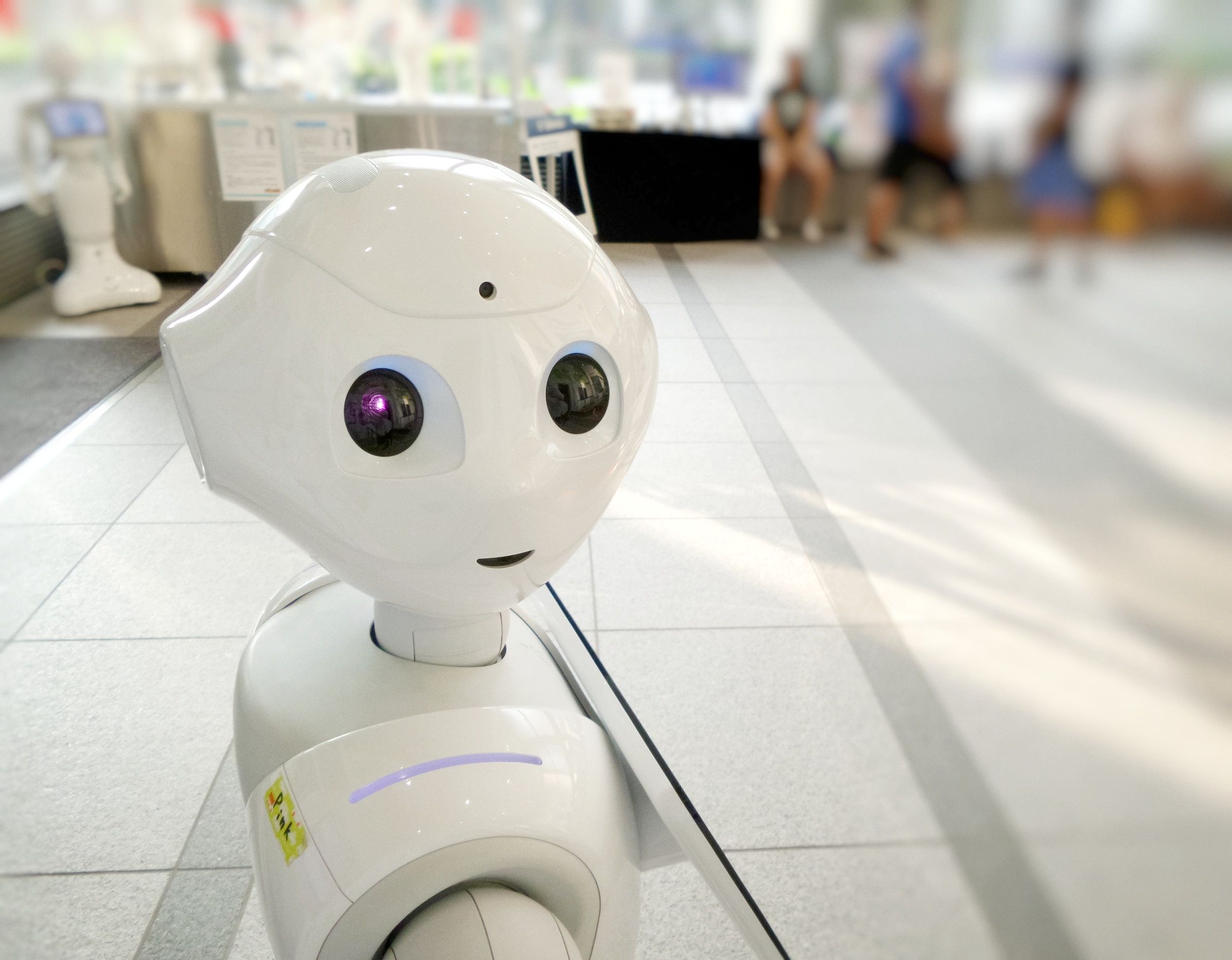
My Experience With OPENAI
April 30, 2023
Want to learn more on harnessing the power of OPENAI's GPT model? SUMM△RISE can summarise any lengthy article in an instant, all thanks to the Article Extractor and Summarizer API. Created using Redux, GPT's article summary API, React and Tailwind.
SUMM△RISE: An AI Powered Article Summariser
Are you tired of reading long and convoluted articles online? Do you find yourself skimming through pages of text, struggling to extract the main points? Look no further! This new project utilizes the power of GPT-based Article Extractor and Summarizer API to provide you with concise summaries of any online article. Go check out the deployed site here!
This project uses cutting-edge technologies such as React.js, Redux Toolkit, and TailwindCSS to create a streamlined user experience that is both easy to use and visually appealing. By leveraging the powerful tools provided by Redux Toolkit, we can fetch data from the Article Extractor and Summarizer API with ease, all while maintaining a clean and organized codebase.
Whether you're a student trying to digest a research paper, a busy professional trying to stay up-to-date on industry news, or just someone who wants to save time while browsing the web, this is the solution you've been searching for. With just a few clicks, you can extract and summarize any article, making it easy to get the information you need in a fraction of the time.
Technologies Used This Week:
- React.js => A JavaScript library for building user interfaces
- Redux => A library used to manage state in react applications
- TailwindCSS => A utility-first CSS framework for quickly building custom user interfaces
- RapidAPI => A platform for building and consuming APIs
As I have spoken at length about Tailwind and React in the previous blogs, my focus here will be my personal experience on using redux toolkit in the context of the Article Extractor and Summariser API. Here is the code used in my project:
store.js
import { configureStore } from '@reduxjs/toolkit';
import { articleApi } from './article';
export const store = configureStore({
reducer: {
[articleApi.reducerPath]: articleApi.reducer,
},
middleware: (getDefaultMiddleware) => getDefaultMiddleware().concat(articleApi.middleware),
})
article.js
import { createApi, fetchBaseQuery } from '@reduxjs/toolkit/query/react'
const rapidApiKey = import.meta.env.VITE_RAPID_API_KEY
export const articleApi = createApi({
reducerPath: 'articleApi',
baseQuery: fetchBaseQuery({
baseUrl: 'https://article-extractor-and-summarizer.p.rapidapi.com',
prepareHeaders: (headers) => {
headers.set('X-RapidAPI-Key', rapidApiKey),
headers.set('X-RapidAPI-Host', 'article-extractor-and-summarizer.p.rapidapi.com')
return headers;
}
}),
endpoints: (builder) => ({
getSummary: builder.query({
query: (params) => `/summarize?url=${encodeURIComponent(params.articleUrl)}&length=3`
})
})
})
export const { useLazyGetSummaryQuery } = articleApi;
In this part of the project, we define and configure our Redux Toolkit API slice for the Article Extractor and Summariser API. This slice, named articleApi
, is created using the createApi
function from the @reduxjs/toolkit/query
package. We start by defining the baseQuery
object, which specifies the base URL and the default headers for our API requests. In this case, we use the fetchBaseQuery
function to create a default query function that uses the RapidAPI key that we stored in our .env
file to authenticate our requests.
Next, we define our API endpoints using the endpoints
function. In this project, we only have one endpoint, which is the getSummary
query. This query takes a single parameter, params.articleUrl
, which represents the URL of the article that we want to summarise.
Finally, we export a hook named useLazyGetSummaryQuery
, which is generated automatically by Redux Toolkit. This hook can be used in our React components to fetch data from the Article Extractor and Summariser API. Overall, using Redux Toolkit API slice and useLazyGetSummaryQuery
hook makes it easy to fetch data from the Article Extractor and Summariser API in a React application. By defining our API endpoints and configuring our baseQuery
object, we can easily create queries and manage their state with the provided hooks. This approach results in a clean and organised codebase, making it easier to maintain and scale the application.
Example of How to Use SUMM△RISE
If you want to see an example of how this project functions, check out this article on Medium: How to be great at asking coding questions, written by Gordon Zhu. As you will probably realise after giving it a quick skim through, this article is rather lengthy indeed! With the power of SUMM△RISE, thousands of words can be reduced to a couple of paragraphs. When the article URL is pasted into the input field, that specific URL is saved in local storage and after a few seconds, the summary is displayed underneath. Here is an example of the article's summary:
Plans for Future Projects
Although some aspects were definitely challenging, I had a great experience working on this project - I had a blast working with the article summariser API and gained a lot of valuable experience in the process! While I am keen to expand my knowledge of redux, I want to attempt to create another Next.js 13 project prior to doing so. To that end, I find myself drawn towards the implementation of AI within a Next.js 13 web app; my next objective is to create a customer support bot, capable of providing quick, efficient assistance to customers with any query or concern they may have.